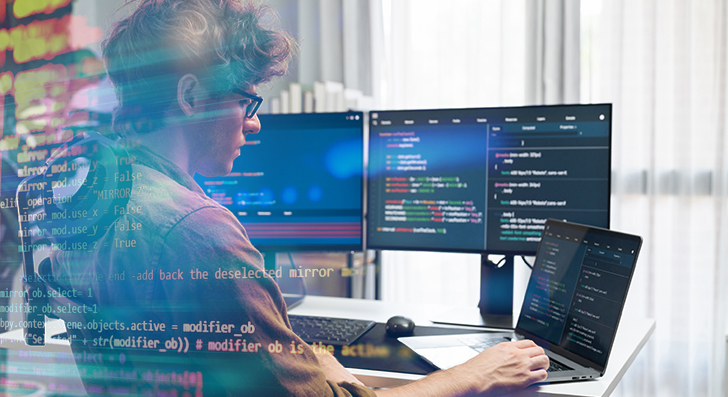
Scalability suggests your software can cope with expansion—a lot more customers, much more facts, plus much more targeted traffic—without having breaking. As a developer, setting up with scalability in mind will save time and pressure later. Right here’s a transparent and useful guide to assist you to start off by Gustavo Woltmann.
Style for Scalability from the beginning
Scalability is not a little something you bolt on afterwards—it should be section of the approach from the beginning. A lot of applications fall short when they increase quickly because the initial structure can’t manage the additional load. As being a developer, you'll want to Believe early regarding how your technique will behave stressed.
Begin by building your architecture to become versatile. Avoid monolithic codebases in which all the things is tightly connected. Alternatively, use modular design and style or microservices. These designs crack your app into smaller sized, impartial pieces. Every module or provider can scale By itself without impacting The complete system.
Also, take into consideration your databases from working day a person. Will it require to deal with 1,000,000 people or simply a hundred? Select the appropriate style—relational or NoSQL—based on how your information will expand. System for sharding, indexing, and backups early, Even when you don’t need to have them still.
A further important stage is to prevent hardcoding assumptions. Don’t compose code that only performs underneath present-day disorders. Take into consideration what would take place When your consumer base doubled tomorrow. Would your app crash? Would the database decelerate?
Use design styles that aid scaling, like information queues or event-driven units. These assistance your application cope with additional requests devoid of finding overloaded.
If you Create with scalability in your mind, you are not just getting ready for success—you're lessening upcoming complications. A properly-planned method is simpler to maintain, adapt, and grow. It’s improved to get ready early than to rebuild later on.
Use the proper Databases
Picking out the appropriate database is a key Element of making scalable programs. Not all databases are constructed a similar, and utilizing the Incorrect you can sluggish you down or even induce failures as your application grows.
Start off by comprehending your details. Could it be highly structured, like rows in a very table? If Certainly, a relational database like PostgreSQL or MySQL is a good healthy. These are definitely sturdy with relationships, transactions, and regularity. In addition they assist scaling methods like examine replicas, indexing, and partitioning to deal with more website traffic and knowledge.
In case your details is more versatile—like user action logs, products catalogs, or paperwork—take into consideration a NoSQL choice like MongoDB, Cassandra, or DynamoDB. NoSQL databases are superior at handling significant volumes of unstructured or semi-structured info and will scale horizontally much more quickly.
Also, consider your read through and generate patterns. Do you think you're doing a lot of reads with fewer writes? Use caching and browse replicas. Will you be handling a large publish load? Take a look at databases that may take care of superior write throughput, and even event-primarily based knowledge storage devices like Apache Kafka (for non permanent information streams).
It’s also wise to Consider in advance. You might not require Superior scaling capabilities now, but choosing a database that supports them implies you gained’t need to have to change later on.
Use indexing to hurry up queries. Prevent avoidable joins. Normalize or denormalize your details depending on your access designs. And often keep an eye on databases functionality while you increase.
Briefly, the appropriate databases is dependent upon your application’s construction, velocity desires, And just how you be expecting it to increase. Just take time to choose properly—it’ll conserve lots of difficulty later.
Improve Code and Queries
Fast code is essential to scalability. As your application grows, every single modest delay adds up. Improperly published code or unoptimized queries can decelerate functionality and overload your program. That’s why it’s vital that you Develop productive logic from the start.
Begin by crafting cleanse, basic code. Stay away from repeating logic and remove just about anything unwanted. Don’t select the most complex Option if a straightforward one particular operates. Keep your features brief, concentrated, and simple to test. Use profiling instruments to discover bottlenecks—places wherever your code will take also long to operate or utilizes far too much memory.
Following, take a look at your databases queries. These frequently sluggish issues down in excess of the code itself. Ensure that Each individual query only asks for the info you actually have to have. Stay away from Find *, which fetches every little thing, and instead pick specific fields. Use indexes to speed up lookups. And stay clear of carrying out too many joins, Specifically throughout big tables.
When you notice precisely the same details becoming asked for many times, use caching. Shop the final results quickly utilizing applications like Redis or Memcached so that you don’t really need to repeat highly-priced operations.
Also, batch your database operations if you can. In lieu of updating a row one by one, update them in groups. This cuts down on overhead and helps make your application additional economical.
Remember to take a look at with significant datasets. Code and queries that work fantastic with one hundred data could crash when they have to handle 1 million.
In brief, scalable apps are quickly apps. Keep the code limited, your queries lean, and use caching when desired. These steps assist your application stay smooth and responsive, even as the load raises.
Leverage Load Balancing and Caching
As your app grows, it has to deal with a lot more consumers and a lot more traffic. If everything goes through one server, it will rapidly become a bottleneck. That’s where load balancing and caching are available. Both of these instruments support maintain your app quick, stable, and scalable.
Load balancing spreads incoming site visitors across multiple servers. Instead of a person server accomplishing the many operate, the load balancer routes consumers to distinct servers according to availability. This means no one server will get overloaded. If 1 server goes down, the load balancer can mail visitors to the Some others. Applications like Nginx, HAProxy, or cloud-dependent answers from AWS and Google Cloud make this easy to arrange.
Caching is about storing facts temporarily so it can be reused promptly. When consumers request the exact same data again—like a product site or even a profile—you don’t have to fetch it within the database every time. You may serve it with the cache.
There are two common sorts of caching:
1. Server-facet caching (like Redis or Memcached) retailers details in memory for speedy accessibility.
two. Client-facet caching (like browser caching or CDN caching) shops static documents close to the consumer.
Caching reduces database load, increases speed, and will make your app additional efficient.
Use caching for things which don’t modify normally. And often be certain your cache is up to date when facts does change.
In a nutshell, load balancing and caching are very simple but effective applications. Together, they help your application handle a lot more people, stay rapid, and recover from difficulties. If you propose to grow, you will need both equally.
Use Cloud and Container Tools
To construct scalable programs, you require applications that let your app expand quickly. That’s where cloud platforms and containers come in. They offer you adaptability, reduce setup time, and make scaling Significantly smoother.
Cloud platforms like Amazon World wide web Expert services (AWS), Google Cloud System (GCP), and Microsoft Azure Permit you to hire servers and services as you will need them. You don’t really need to read more obtain components or guess long run potential. When targeted visitors increases, you'll be able to incorporate far more methods with just a couple clicks or mechanically working with car-scaling. When website traffic drops, you may scale down to save cash.
These platforms also offer you companies like managed databases, storage, load balancing, and protection equipment. You'll be able to give attention to creating your app instead of handling infrastructure.
Containers are Yet another important tool. A container offers your application and every little thing it has to run—code, libraries, configurations—into just one unit. This makes it easy to maneuver your app between environments, from a laptop computer for the cloud, with out surprises. Docker is the most popular Software for this.
Once your app uses many containers, equipment like Kubernetes assist you to manage them. Kubernetes handles deployment, scaling, and Restoration. If a single part of your respective app crashes, it restarts it automatically.
Containers also help it become simple to different areas of your app into expert services. You may update or scale elements independently, which is great for performance and dependability.
In short, working with cloud and container resources usually means you'll be able to scale speedy, deploy simply, and recover speedily when issues transpire. If you would like your application to grow with no restrictions, start out utilizing these instruments early. They save time, minimize hazard, and enable you to keep centered on developing, not repairing.
Observe Every little thing
When you don’t monitor your application, you gained’t know when points go wrong. Monitoring will help the thing is how your application is carrying out, location issues early, and make far better selections as your application grows. It’s a vital A part of constructing scalable devices.
Get started by tracking fundamental metrics like CPU utilization, memory, disk Room, and reaction time. These inform you how your servers and products and services are performing. Resources like Prometheus, Grafana, Datadog, or New Relic can assist you accumulate and visualize this facts.
Don’t just observe your servers—monitor your app also. Control just how long it will require for people to load web pages, how frequently glitches transpire, and wherever they manifest. Logging applications like ELK Stack (Elasticsearch, Logstash, Kibana) or Loggly will let you see what’s going on within your code.
Build alerts for significant challenges. One example is, If the reaction time goes earlier mentioned a limit or even a support goes down, you ought to get notified quickly. This will help you resolve problems quick, often right before buyers even detect.
Monitoring can also be useful after you make improvements. When you deploy a whole new characteristic and see a spike in glitches or slowdowns, it is possible to roll it back before it will cause true harm.
As your application grows, targeted traffic and info boost. Without checking, you’ll skip indications of difficulties till it’s much too late. But with the best resources set up, you remain on top of things.
In a nutshell, checking aids you keep your app reliable and scalable. It’s not almost spotting failures—it’s about comprehension your method and ensuring that it works very well, even under pressure.
Ultimate Thoughts
Scalability isn’t just for large providers. Even tiny applications require a robust Basis. By developing diligently, optimizing properly, and utilizing the right equipment, you can Create applications that develop efficiently without breaking under pressure. Start out small, Feel significant, and Develop sensible.